WordPress の関数にサイトの URL を取得する関数がいくつかある。get_home_url()
、home_url()
、get_site_url()
、site_url()
等。プラグインやテーマを開発するなら、違いを把握しておいた方が良い。ということで、混乱しがちなこれらの関数の違いを整理しておこう。
結論から言うと、プログラムが内部で、必要なファイルのパスからそのファイルの URL を割り出したりするのに必要なのが、get_site_url()
で、エンドユーザーが「自分のホームページってここなの!」ってみんなにサイトのホームとして晒せたい場所が get_home_url()
。どういうことか見ていこう。
一般設定
まず、設定にサイトの URL を指定する箇所があるのを確認。
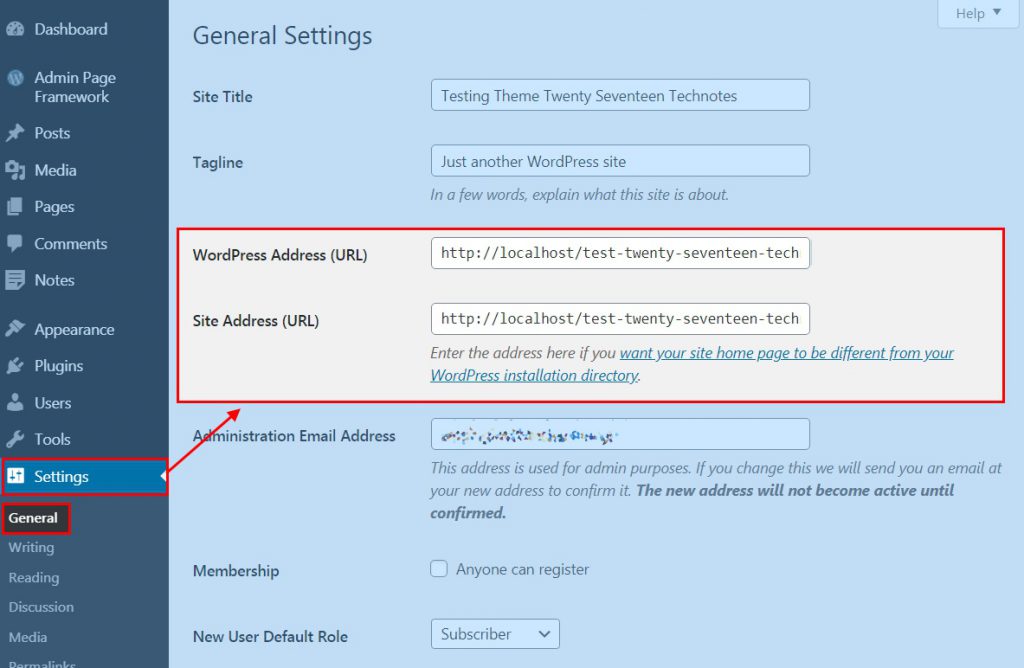
で、オプションのキーの名称も確認。
キー名
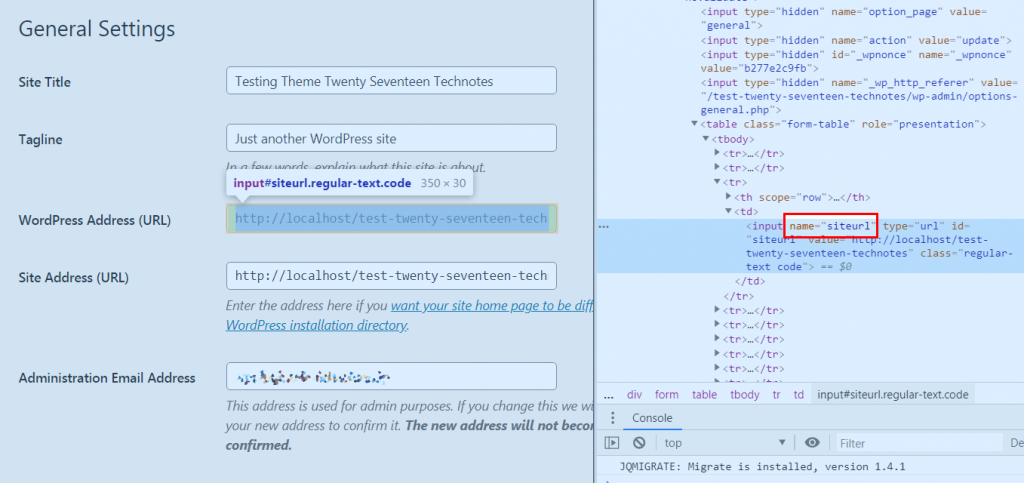
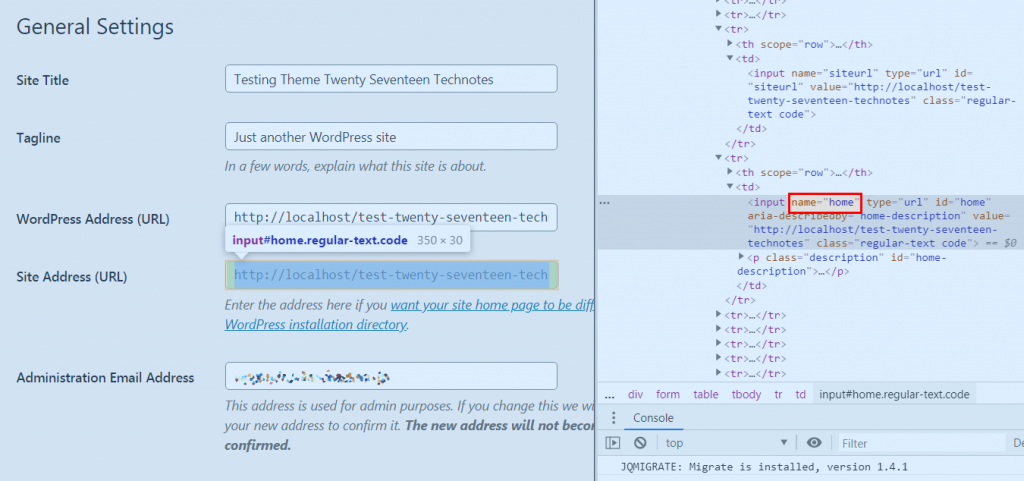
WordPress Address が siteurl で Site Address が home というキー名になっている。
オプションテーブル
これらがデータベースでどのように格納されているかも確認しておこう。
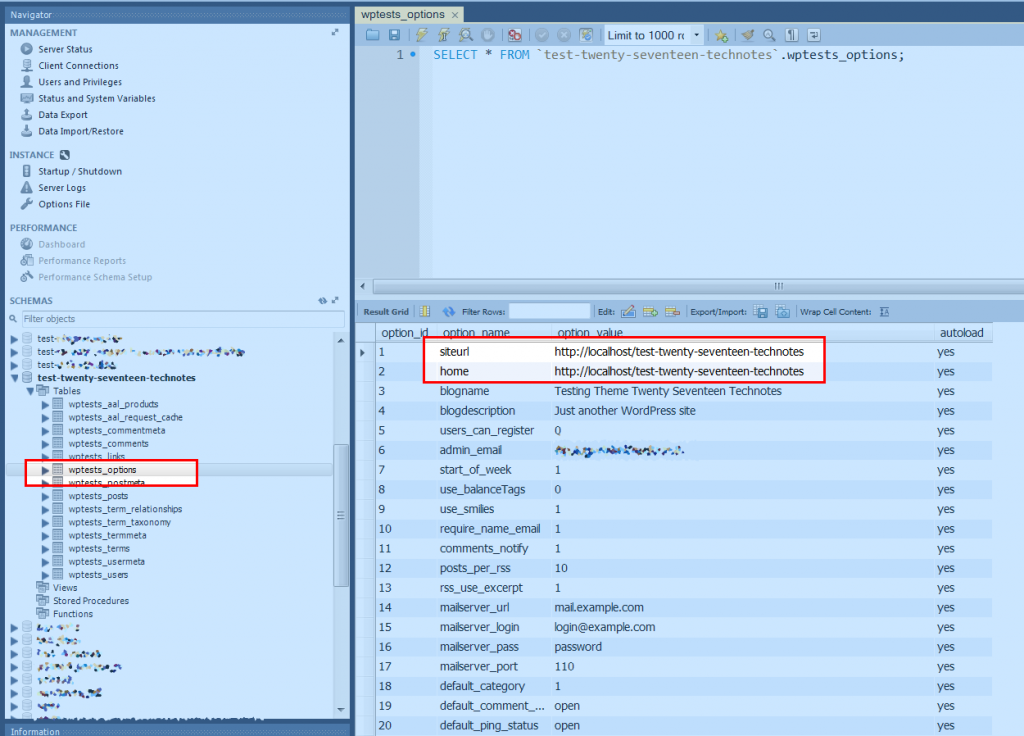
{table name prefix}_options
テーブル の option_name
カラムの siteurl
と home
キーに option_value
として入力した URL が格納されていることがわかる。
PHP コード
次に、コードを見ていこう。
home_url()
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
/** * Retrieves the URL for the current site where the front end is accessible. * * Returns the 'home' option with the appropriate protocol. The protocol will be 'https' * if is_ssl() evaluates to true; otherwise, it will be the same as the 'home' option. * If `$scheme` is 'http' or 'https', is_ssl() is overridden. * * @since 3.0.0 * * @param string $path Optional. Path relative to the home URL. Default empty. * @param string|null $scheme Optional. Scheme to give the home URL context. Accepts * 'http', 'https', 'relative', 'rest', or null. Default null. * @return string Home URL link with optional path appended. */ function home_url( $path = '', $scheme = null ) { return get_home_url( null, $path, $scheme ); } |
get_home_url()
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 |
/** * Retrieves the URL for a given site where the front end is accessible. * * Returns the 'home' option with the appropriate protocol. The protocol will be 'https' * if is_ssl() evaluates to true; otherwise, it will be the same as the 'home' option. * If `$scheme` is 'http' or 'https', is_ssl() is overridden. * * @since 3.0.0 * * @global string $pagenow * * @param int $blog_id Optional. Site ID. Default null (current site). * @param string $path Optional. Path relative to the home URL. Default empty. * @param string|null $scheme Optional. Scheme to give the home URL context. Accepts * 'http', 'https', 'relative', 'rest', or null. Default null. * @return string Home URL link with optional path appended. */ function get_home_url( $blog_id = null, $path = '', $scheme = null ) { global $pagenow; $orig_scheme = $scheme; if ( empty( $blog_id ) || ! is_multisite() ) { $url = get_option( 'home' ); } else { switch_to_blog( $blog_id ); $url = get_option( 'home' ); restore_current_blog(); } if ( ! in_array( $scheme, array( 'http', 'https', 'relative' ) ) ) { if ( is_ssl() && ! is_admin() && 'wp-login.php' !== $pagenow ) { $scheme = 'https'; } else { $scheme = parse_url( $url, PHP_URL_SCHEME ); } } $url = set_url_scheme( $url, $scheme ); if ( $path && is_string( $path ) ) { $url .= '/' . ltrim( $path, '/' ); } /** * Filters the home URL. * * @since 3.0.0 * * @param string $url The complete home URL including scheme and path. * @param string $path Path relative to the home URL. Blank string if no path is specified. * @param string|null $orig_scheme Scheme to give the home URL context. Accepts 'http', 'https', * 'relative', 'rest', or null. * @param int|null $blog_id Site ID, or null for the current site. */ return apply_filters( 'home_url', $url, $path, $orig_scheme, $blog_id ); } |
site_url()
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
/** * Retrieves the URL for the current site where WordPress application files * (e.g. wp-blog-header.php or the wp-admin/ folder) are accessible. * * Returns the 'site_url' option with the appropriate protocol, 'https' if * is_ssl() and 'http' otherwise. If $scheme is 'http' or 'https', is_ssl() is * overridden. * * @since 3.0.0 * * @param string $path Optional. Path relative to the site URL. Default empty. * @param string $scheme Optional. Scheme to give the site URL context. See set_url_scheme(). * @return string Site URL link with optional path appended. */ function site_url( $path = '', $scheme = null ) { return get_site_url( null, $path, $scheme ); } |
get_site_url()
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 |
/** * Retrieves the URL for a given site where WordPress application files * (e.g. wp-blog-header.php or the wp-admin/ folder) are accessible. * * Returns the 'site_url' option with the appropriate protocol, 'https' if * is_ssl() and 'http' otherwise. If `$scheme` is 'http' or 'https', * `is_ssl()` is overridden. * * @since 3.0.0 * * @param int $blog_id Optional. Site ID. Default null (current site). * @param string $path Optional. Path relative to the site URL. Default empty. * @param string $scheme Optional. Scheme to give the site URL context. Accepts * 'http', 'https', 'login', 'login_post', 'admin', or * 'relative'. Default null. * @return string Site URL link with optional path appended. */ function get_site_url( $blog_id = null, $path = '', $scheme = null ) { if ( empty( $blog_id ) || ! is_multisite() ) { $url = get_option( 'siteurl' ); } else { switch_to_blog( $blog_id ); $url = get_option( 'siteurl' ); restore_current_blog(); } $url = set_url_scheme( $url, $scheme ); if ( $path && is_string( $path ) ) { $url .= '/' . ltrim( $path, '/' ); } /** * Filters the site URL. * * @since 2.7.0 * * @param string $url The complete site URL including scheme and path. * @param string $path Path relative to the site URL. Blank string if no path is specified. * @param string|null $scheme Scheme to give the site URL context. Accepts 'http', 'https', 'login', * 'login_post', 'admin', 'relative' or null. * @param int|null $blog_id Site ID, or null for the current site. */ return apply_filters( 'site_url', $url, $path, $scheme, $blog_id ); } |
違い
home_url()
は get_home_url()
を、site_url()
は get_site_url()
をそれぞれ呼び出しているので、
- home_url() と get_home_url()
- site_url() と get_site_url()
大まかにこの2種類があると考えて良い。先に確認したオプションキーも2つだった。そして、それぞれ
get_home_url()
はget_option( 'home' )
get_site_url()
はget_option( 'siteurl' )
というラインがある。
ここまでだと、どちらもユーザーが設定したURLを参照してるに過ぎない。そこで、plugins_url()
の中身を見ると、WP_PLUGIN_URL
という定数を参照している。そしてその定数は、WP_CONTENT_URL
を参照している。そしてそれは、
1 2 3 4 |
function wp_plugin_directory_constants() { if ( ! defined( 'WP_CONTENT_URL' ) ) { define( 'WP_CONTENT_URL', get_option( 'siteurl' ) . '/wp-content' ); // full url - WP_CONTENT_DIR is defined further up } |
とあるように、siteurl
の値を取得している。
また、get_template_directory_uri()
の中身を見ると、get_theme_root_uri()
が使用され、それは content_url()
、site_url()
、plugins_url()
を使用している。content_url()
を見ると、plugins_url()
ど同様に WP_CONTENT_URL
を参照している。
まとめ
つまり、plugins_url()
や get_theme_directory_uri()
などのプログラムが内部でパスを割り出す為に参照するのは siteurl
オプションの値となっている。
一方、get_home_url()
もしくは home_url()
はテーマ(テンプレート)を扱うときに、エンドユーザーが指定した場所へリンクを生成する時などに使われている。ユーザーがポートフォリオのページを別途作っており、そこをホームページにしたい、といったケースで Site Address のオプションは設定され、 get_home_url()
及び home_url()
はその home
オプションに格納された値を参照する。
参考:https://wordpress.stackexchange.com/questions/20294/whats-the-difference-between-home-url-and-site-url